Are you tired of waiting for your web application to load? Are you tired of watching your screen freeze up every time you try to interact with it? Change detection is the answer! But which framework has the better change detection mechanism, Angular or React? Let’s find out.
What is Change Detection?
Change detection is the process by which a framework or library detects changes to data and updates the user interface accordingly. In the context of web development, this typically refers to the process of updating the user interface in response to changes in the application state or user input.
Angular Change Detection

Angular uses a change detection mechanism that works by traversing the component tree, starting from the root component, and checking each component and its child components for changes.
Angular uses a Zone.js library to implement change detection. Zones provide a way to intercept and track asynchronous operations, including those triggered by browser events, and trigger change detection as needed. Angular’s change detection algorithm works by traversing the component tree, starting from the root component, and checking each component and its child components for changes.
By default, Angular’s change detection runs every time an event occurs, which can result in performance issues in large and complex applications. To optimize performance, developers can use the on-push change detection strategy, which runs change detection only when the input properties of a component change or when an event is triggered by the component itself.
To use the on-push change detection strategy, you need to change the change detection mode of a component from default to on-push. This strategy requires careful management of the component state, and developers must ensure that all input properties of a component are immutable and that any changes to the state are made using pure functions. This can be done in the component decorator by adding the changeDetection
property with the value of ChangeDetectionStrategy.OnPush
, like this:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-todo-component',
template: `
<h1>{{ title }}</h1>
<button (click)="updateTitle()">Update Title</button>
`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ToDoComponent {
title = 'My TO-DO List';
updateTitle() {
this.title = 'My TO-DO List for April 11, 2023';
}
}
In this example, the ToDoComponent
is using the on-push strategy, which means that it will only run change detection when the input properties of the component change or when an event is triggered by the component itself, such as the button click event.
React Change Detection
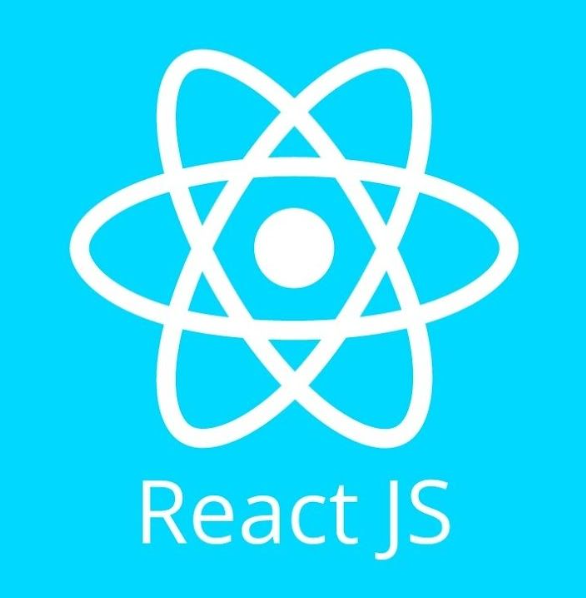
React uses a virtual DOM (VDOM) to implement change detection. When a component’s state or props change, React updates the VDOM and then compares the updated VDOM to the previous VDOM to determine which parts of the UI need to be updated.
React’s VDOM allows it to minimize the number of actual DOM updates required, which can result in better performance compared to Angular. However, the VDOM approach can also introduce overhead, particularly in large and complex applications.
To optimize performance in React, developers can use memoization to prevent unnecessary re-rendering of components. Memoization is a technique that involves caching the results of expensive computations so that they can be reused later without recomputing them. By memoizing expensive computations, developers can reduce the number of times that components need to be re-rendered, improving overall performance.
Here’s an example of memoizing a value in React using the useMemo
hook:
import React, { useState, useMemo } from 'react';
function ExpensiveCalculation() {
console.log('Performing expensive calculation...');
// ... expensive computation here ...
return calculatedValue;
}
function Example() {
const [value, setValue] = useState(0);
const calculatedValue = useMemo(() => ExpensiveCalculation(), [value]);
function handleClick() {
setValue(value + 1);
}
return (
<div>
<button onClick={handleClick}>Increment Value</button>
<div>Calculated Value: {calculatedValue}</div>
</div>
);
}
In this example, ExpensiveCalculation
is a function that performs a computationally expensive calculation and returns a value. In the Example
component, the useMemo
hook is used to memoize the value returned by ExpensiveCalculation
. The useMemo
hook takes two arguments: a function that performs the expensive calculation and an array of dependencies that determine when the calculation should be re-executed. In this case, the dependency is value
, which is updated whenever the user clicks the button.
When the user clicks the button, the value
state is updated, triggering a re-render of the Example
component. However, because the value returned by ExpensiveCalculation
is memoized using useMemo
, the calculation is only re-executed when value
changes. This can significantly improve the performance of the application by reducing the number of unnecessary calculations.
Comparing Angular and React Change Detection
So, which change detection mechanism is better, Angular or React? The answer is, it depends on your application’s requirements. Angular’s change detection is more straightforward and easier to manage but can be less performant in large and complex applications. React’s VDOM approach is more complex but can be more performant by minimizing the number of actual DOM updates required.
To achieve high performance in both Angular and React, developers must carefully manage component state and use techniques such as memoization to optimize rendering. Developers should also be aware of the trade-offs between different change detection approaches and choose the one that best suits their application’s requirements.
To sum it up, Change detection is a critical part of any web application that involves dynamic updates to the user interface. Angular and React provide different mechanisms for implementing change detection, and each has its strengths and weaknesses. By understanding these differences and using best practices for managing component state and optimizing rendering, developers can achieve high performance in both frameworks. So, choose your framework wisely, and happy coding!